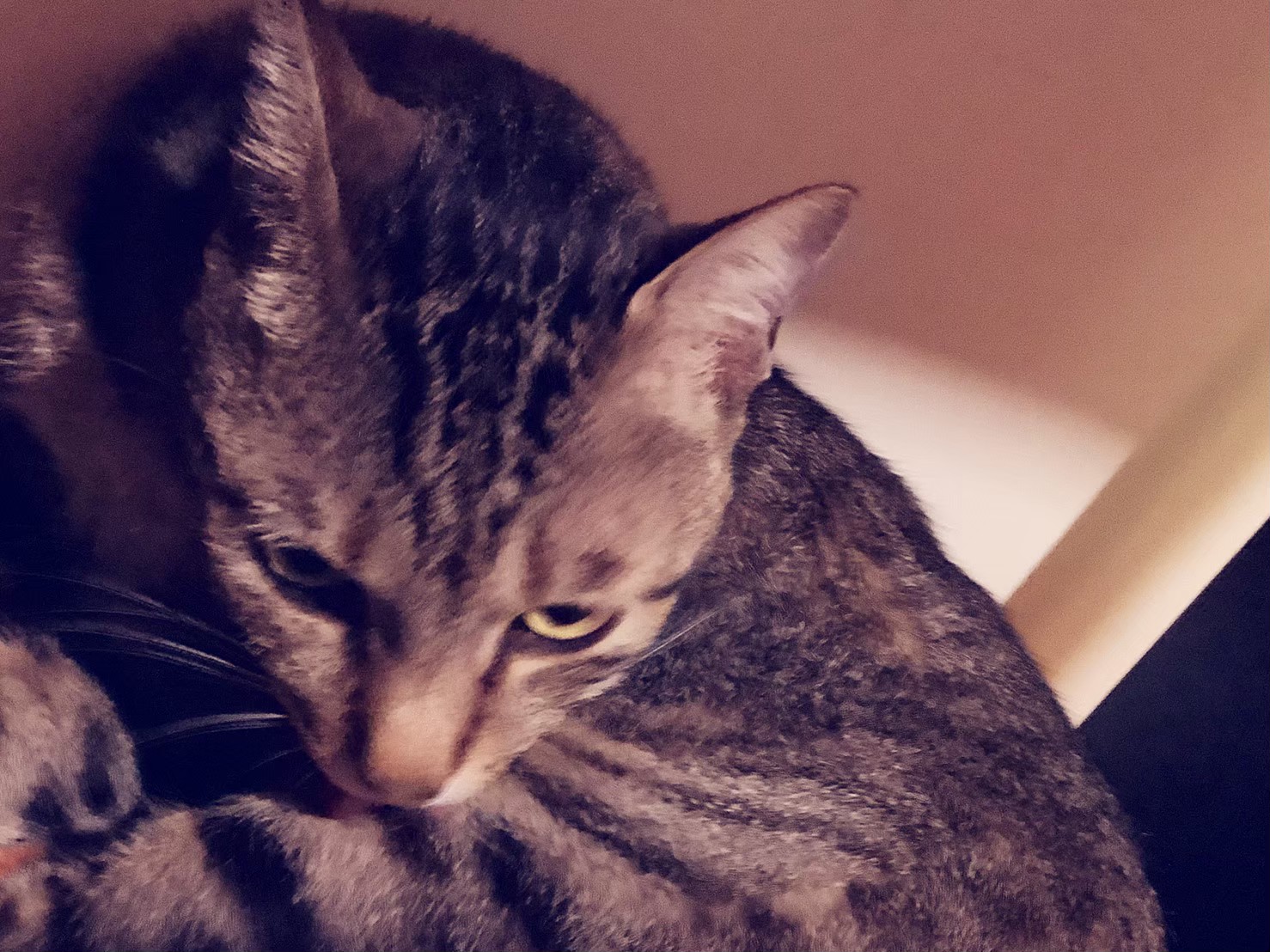
UVA 00572 - Oil Deposits
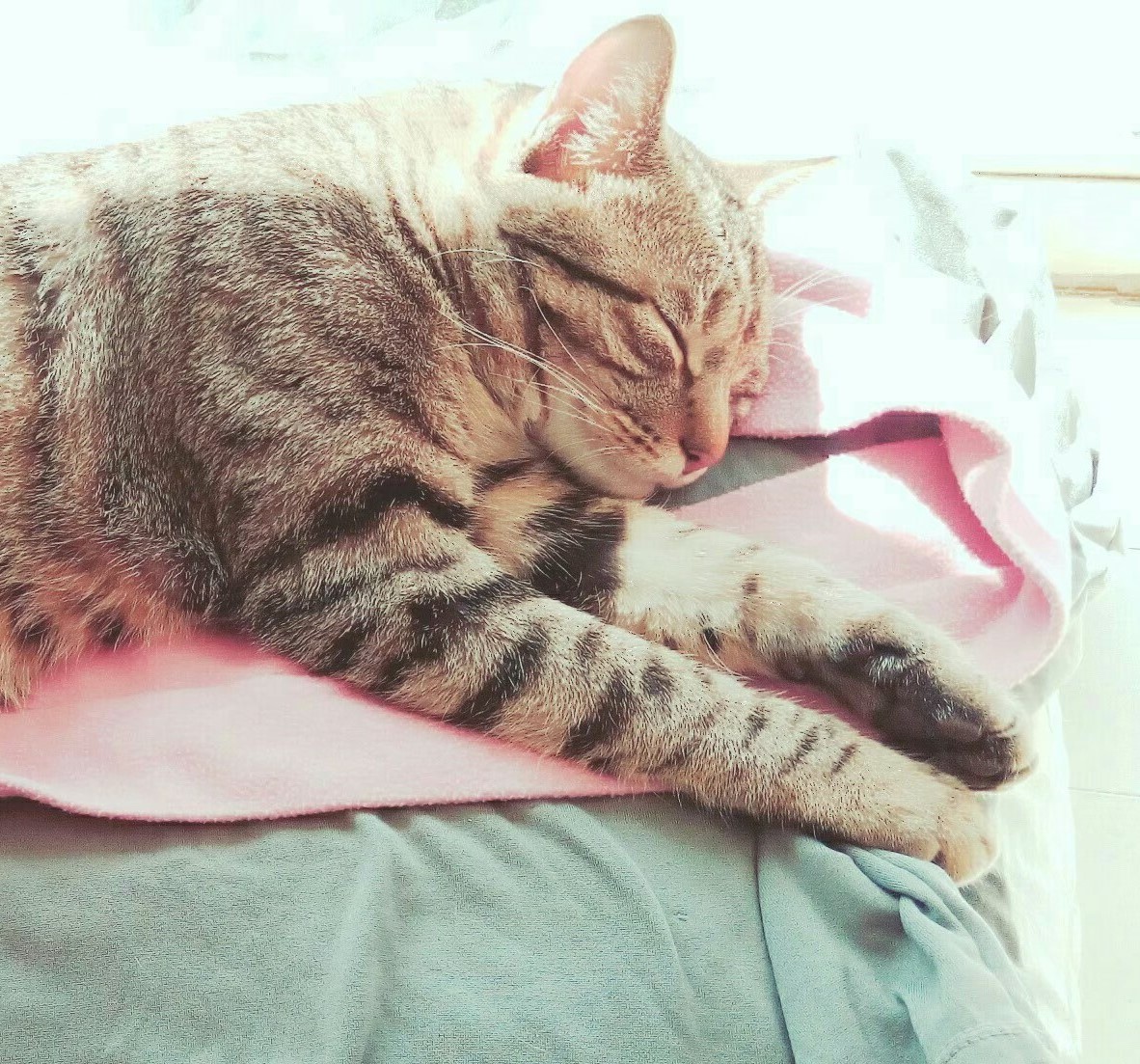
解題觀念
BFS & DFS
可以參考我的課程簡報:BFS & DFS
關於 BFS 的基本觀念可以參考我的這篇文章:Algorithm - BFS
關於 DFS 的基本觀念可以參考我的這篇文章:Algorithm - DFS
題目敘述
有家石油公司負責探勘某塊地底下的石油含量,這塊地是矩行的
含有石油的一小塊地稱作一個 pocket,假如兩個 pocket 相連,則這兩個 pocket 屬於同一個 oil deposit
你的任務就是要找出這塊地包含幾個不同的 oil deposit
Input
輸入包含好幾組資料,每組資料的第一行有 2 個整數 m, n
m 代表這塊地的列數,n代表這塊地的行數(1 <= m, n <= 100)
接下來的 m 行是這塊地探勘的內容@
代表此小塊含石油,*
代表此小塊不含石油
當 m = 0 && n = 0 則輸入結束
1 | // Sample Input |
Output
對每組測試資料輸出 oil deposit 的數目
1 | // Sample Output |
Solution Code
1 |
|