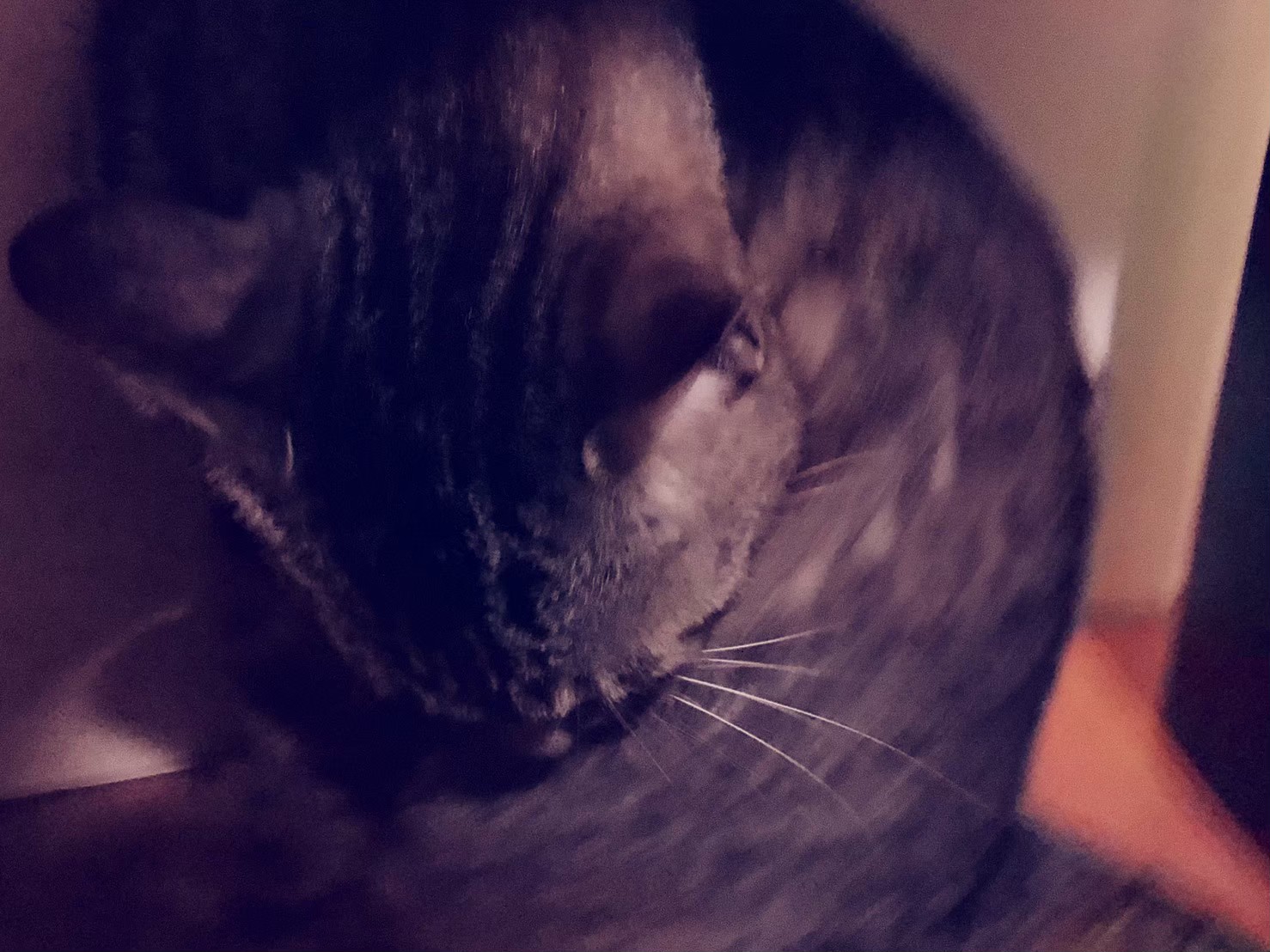
UVA 10189 - Minesweeper
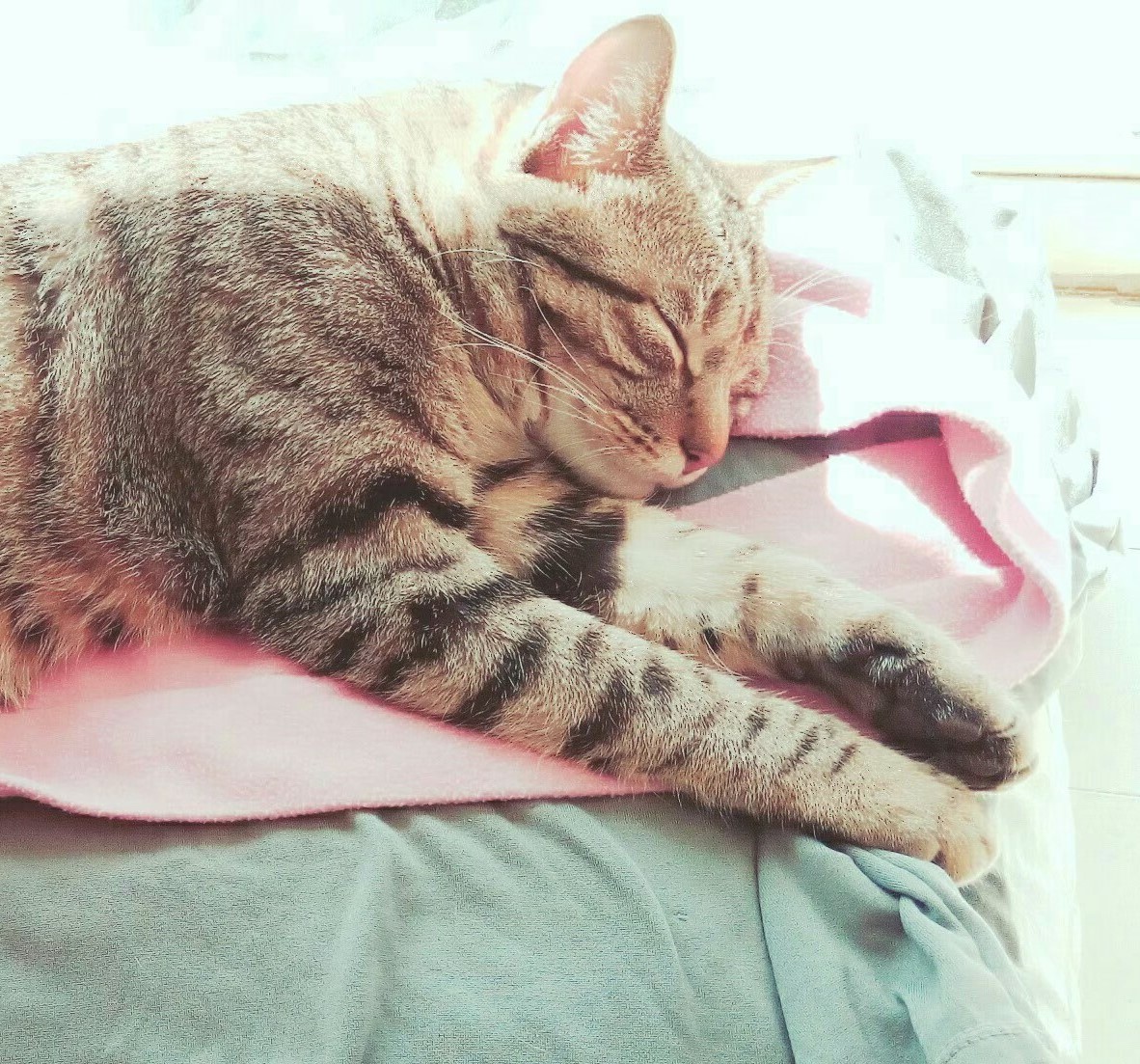
解題觀念
BFS & DFS
可以參考我的課程簡報:BFS & DFS
關於 BFS 的基本觀念可以參考我的這篇文章:Algorithm - BFS
關於 DFS 的基本觀念可以參考我的這篇文章:Algorithm - DFS
題目敘述
您玩過《踩地雷》嗎?這是一款可愛的小遊戲,遊戲的目標是找到所有 M × N 地圖內的地雷
遊戲在一個正方形中顯示一個數字,告訴您該正方形附近有多少個地雷
例如,假設下面的4×4的地圖內帶有 2 個地雷 ( 以 *
字元表示 )
如果我們根據上述作法,將遊戲提示數字填入,則結果將為:
每個正方形內的數字最多為 8 ( 因為最多有8個正方形相鄰 )
1 | // 地雷 |
1 | // 數字帶入周遭有多少地雷 |
Input
輸入將包含多組測資
每組測資第一行包含兩個整數 n 和 m ( 0 < n, m ≤ 100 ),代表地圖大小
如果 n = m = 0 代表輸入結束
接下來的n行,每行m個字元,代表整張地圖
每個安全方塊用 “.” 字元表示,每個地雷方塊用 *
字元表示
1 | // Sample Input |
Output
對於每組測資
輸出第一行為 “Field #k:”,k代表測資編號
接下來輸出題示後的遊戲地圖
每筆測資間請用空白行分隔
1 | // Sample Output |
Solution Code
1 |
|