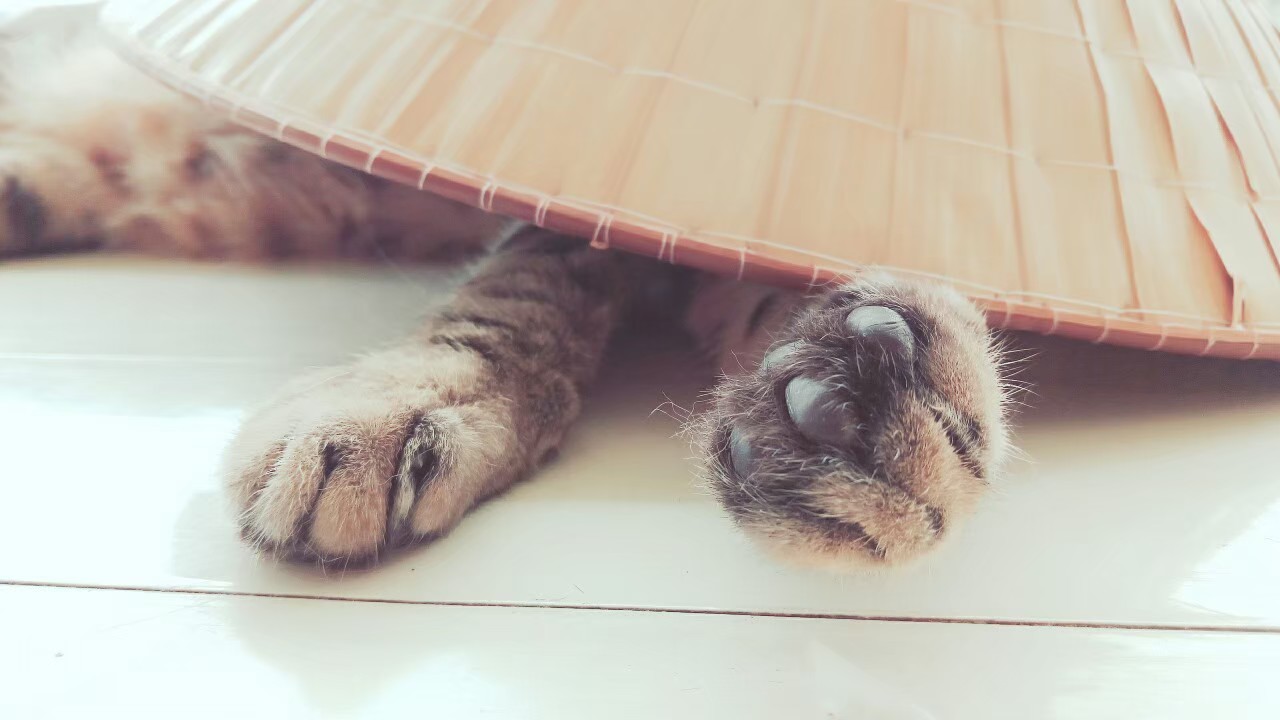
OpenAI API - Connect OpenAI Model with MATLAB Program and Automatically Send Gmail by SMTP
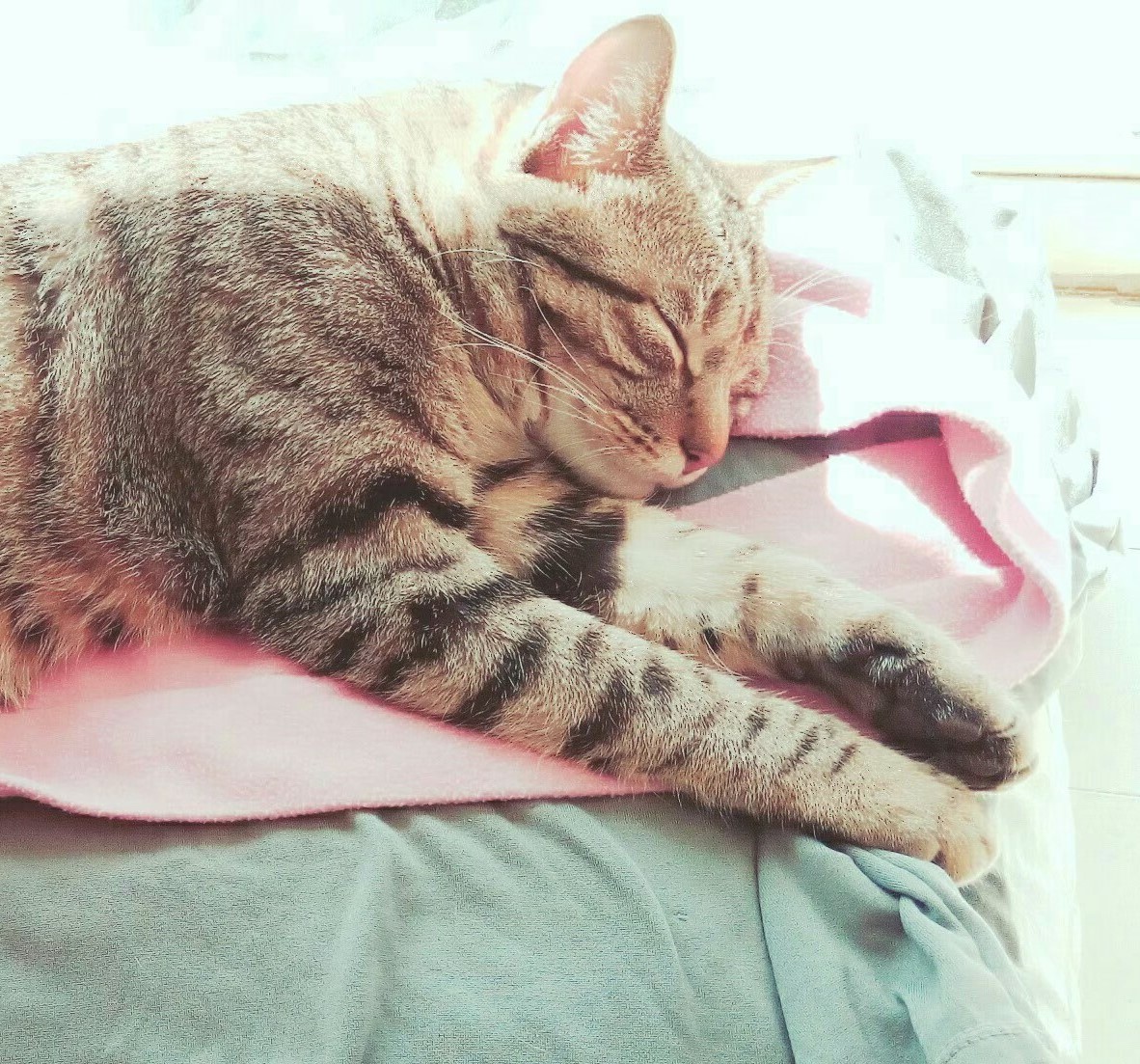
Research Motivation
因 OpenAI 及其旗下的 ChatGPT 目前為資訊界大勢熱門議題
嘗試透過 OpenAI 提供之 API 服務、結合 MATLAB 撰寫程式自動連線對 OpenAI 之 Davinci model 進行 prompt 詢問
並將其 Response 的結果撰寫 MATLAB 程式以 SMTP 協定自動寄信給指定收件人
Teaching Steps PPT
剛好同個學期選修的進階生活美語課程有個人 Mini-Talk Presentation 演講
於是我就拿 OpenAI API with MATLAB Program 當作主題了 XD
所以順道有製作 Presentation PPT
雖然是全英文的,但如果需要理解程式碼和運作原理的話,可以加減參考
What is API
本 side project 主要圍繞在如何應用 OpenAI 的 API 服務
那究竟什麼是 API 呢?
可參考我的這篇文章:
架構

Session 1. 使用 MATLAB 撰寫程式連線 OpenAI API 進行詢問
需先至 OpenAI API 註冊帳號並生成自己的 API key
如何生成 OpenAI API key 可以參考我的這篇文章:
OpenAI API - How to generate OpenAI API keyCode
1 | import matlab.net.* |
幾個小小細節:api_key = convertCharsToStrings(getenv("APIkey"));
:
- 從系統環境變數中提取,為了不讓個人 API key 裸奔在程式碼中,提高安全性
- 因為從系統環境變數中提取的型態是字元,故這裡要再將其轉為字串型態,以符合其連線所需的讀取型別
parameters = struct('prompt',prompt, 'max_tokens',100);
- 設定 model 根據 prompt 而回覆的 response 的最多 tocken 數
- 因為 OpenAI 會根據 response 的 tocken 數計費,
回太多字錢包會燒起來
結果
我向 OpenAI 詢問的問題是 “Please introduce Cloud Computing”
他回覆的結果為:
Computing is raising a lot of eyebrows these days, but what is it really? This site provides more clarity.Cloud Disaster RecoveryCloud is the way of the future, so it is important to develop tools that will protect your data. Come to the Dis2 team to discuss solutions to your data recovery needs.Learn to Make the Most out of Your Microsoft Office SkillsA group of professionals met monthly at the Microsoft Software Center in Voorhees for some training tips..
Session 2. 使用 MATLAB 撰寫程式寄 Gmail 信件至指定收件者的信箱
自從 2022 年 5 月 30 後 Gmail 無法直接使用 SMTP 寄信,需進行二階段驗證
故要寄信的程式需特別開啟對於該應用程式軟體的二階段驗證 + 設定應用程式密碼
Code
1 | % Automatically Sending Gmail |
億點點細節:
- 一些路徑和使用者名稱和信箱要再替換!
- SMTP port 選擇
- 25:標準SMTP埠,並且主要用於SMTP中繼,但可能會因濫用埠 25 從受感染的電腦發送垃圾郵件
- 587:現代網路上 SMTP 提交的默認埠
- 465:最初是為 SMTPS(基於 SSL 的 SMTP)註冊的,現已棄用,但許多 ISP 和雲託管提供商仍支持 465 埠用於 SMTP
password = convertCharsToStrings(getenv("Passwd"));
:
- 把密碼存於環境變數,這裡的密碼是 Google 帳戶應用程式登入密碼
- 如何設定 Google 帳戶應用程式登入密碼請看我的這篇文章: Google - sign in with app passwords
sendmail('<destination>@gmail.com','Introduction to Cloud Computing Homework''The attached file is my Matlab homework Code, Thanks!', ...'See the attached files for more info.',{'<file path>'});
:
- sendmail(收件人信箱, 信件主旨, 信件內文, 附加檔案在本機的路徑)
結果
這是我寄信的信箱的寄件備份,信件實被自動撰寫寄出
Reference
- Connecting to ChatGPT using API
- Math Works - getenv
- Math Works - convertCharsToStrings
- Math Works - Send Email
- MATLAB 使用 sendmail 函數寄 Gmail 信件
- MATLAB 自動寄信功能
- Using gmail after May 30, 2022
- Sign in with app passwords
- How to send an email using MATLAB? How to check internet connection in MATLAB? (YouTube)
- SMTP port 選擇