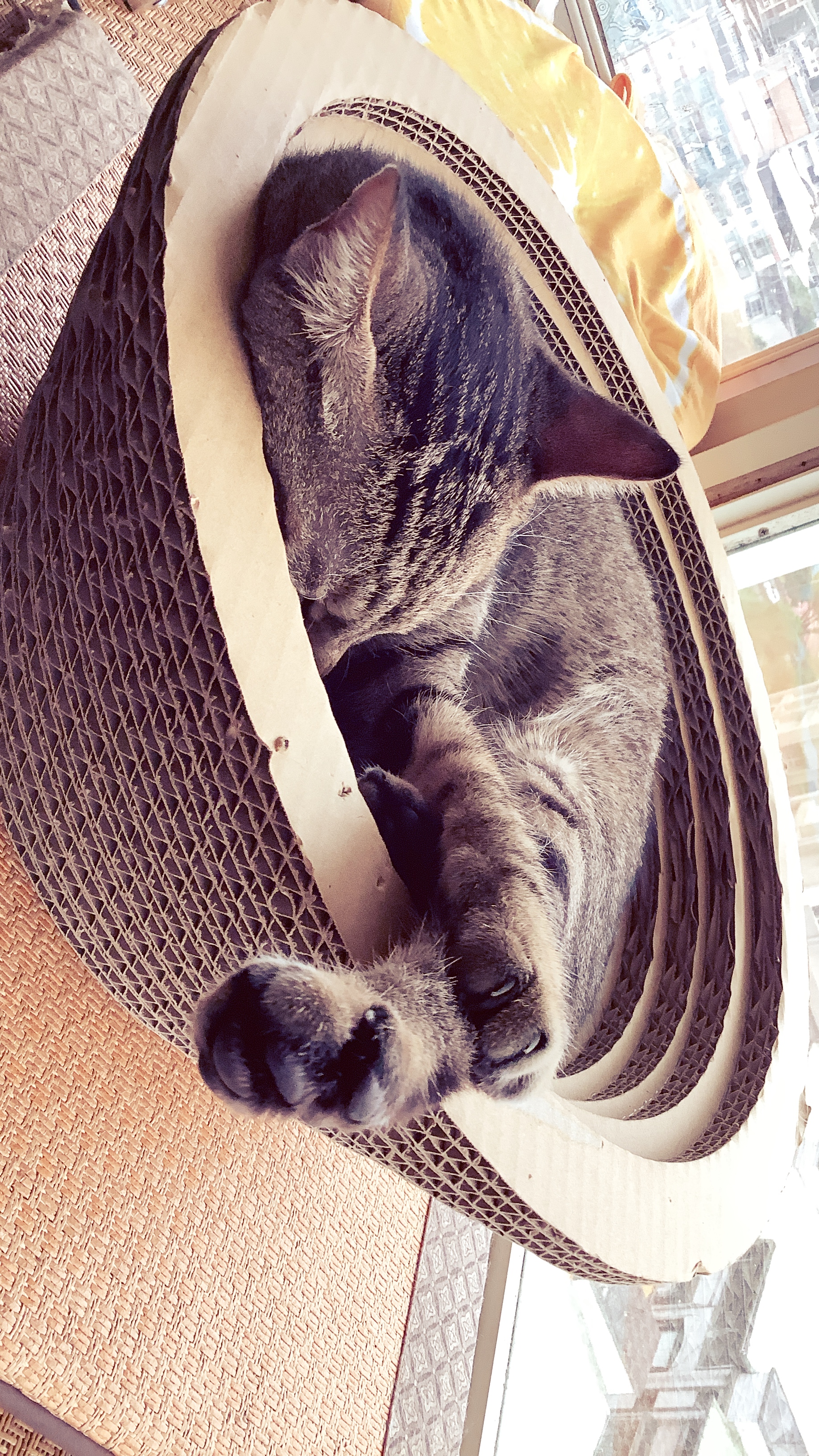
Python - Matplotlib
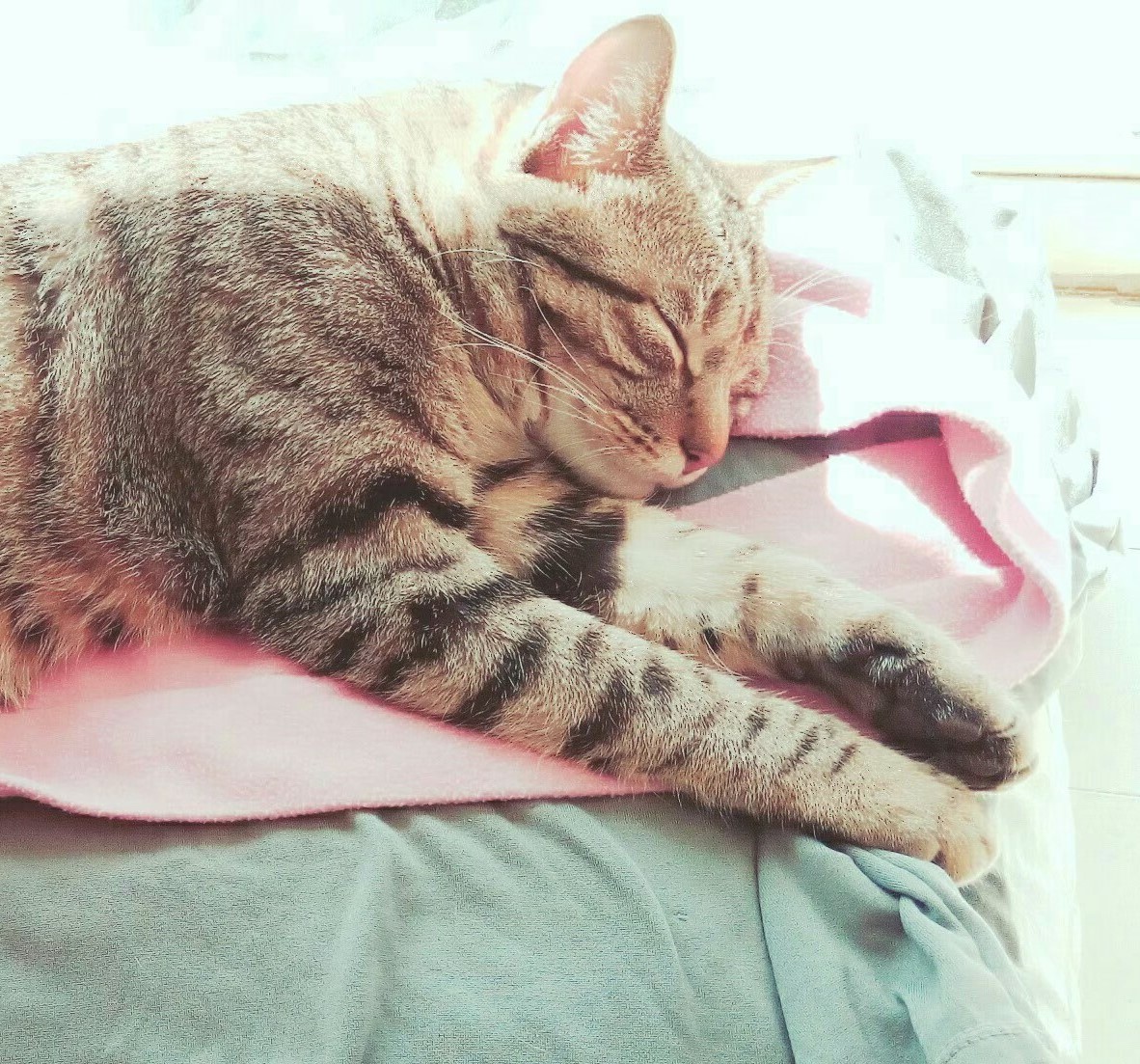
Course
可以參考我的課程簡報:
Python IntroductionMatplotlib 繪製教學
Install
1 | pip install matplotlib |
pyplot
畫折線圖
1 | import matplotlib.pyplot as plt |

setting color
設定線段顏色
1 | import matplotlib.pyplot as plt |

axis label & title
加上圖片標題、x 軸 y 軸名稱
1 | import matplotlib.pyplot as plt |

multiple data
同時要畫多條線的時候
1 | import matplotlib.pyplot as plt |

grid
可以在圖上加上網格
1 | import matplotlib.pyplot as plt |

setp
可以在線段上加點原點,方便標示
1 | import matplotlib.pyplot as plt |

legend
小標籤,可以標示線段、顏色之類的涵義
1 | import matplotlib.pyplot as plt |

Reference
- 瀚丰學長的深度學習課程簡報