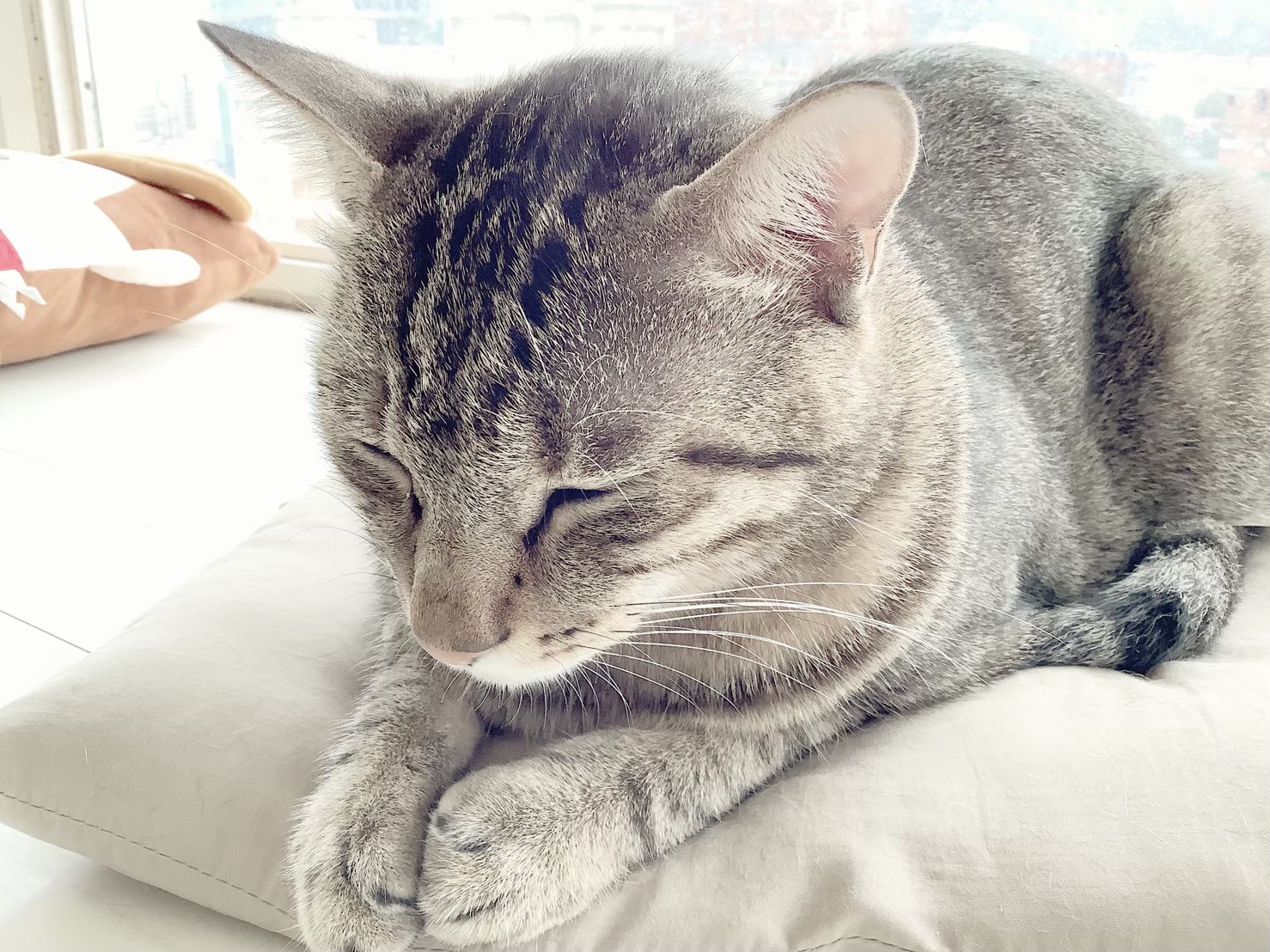
Python - Syntax
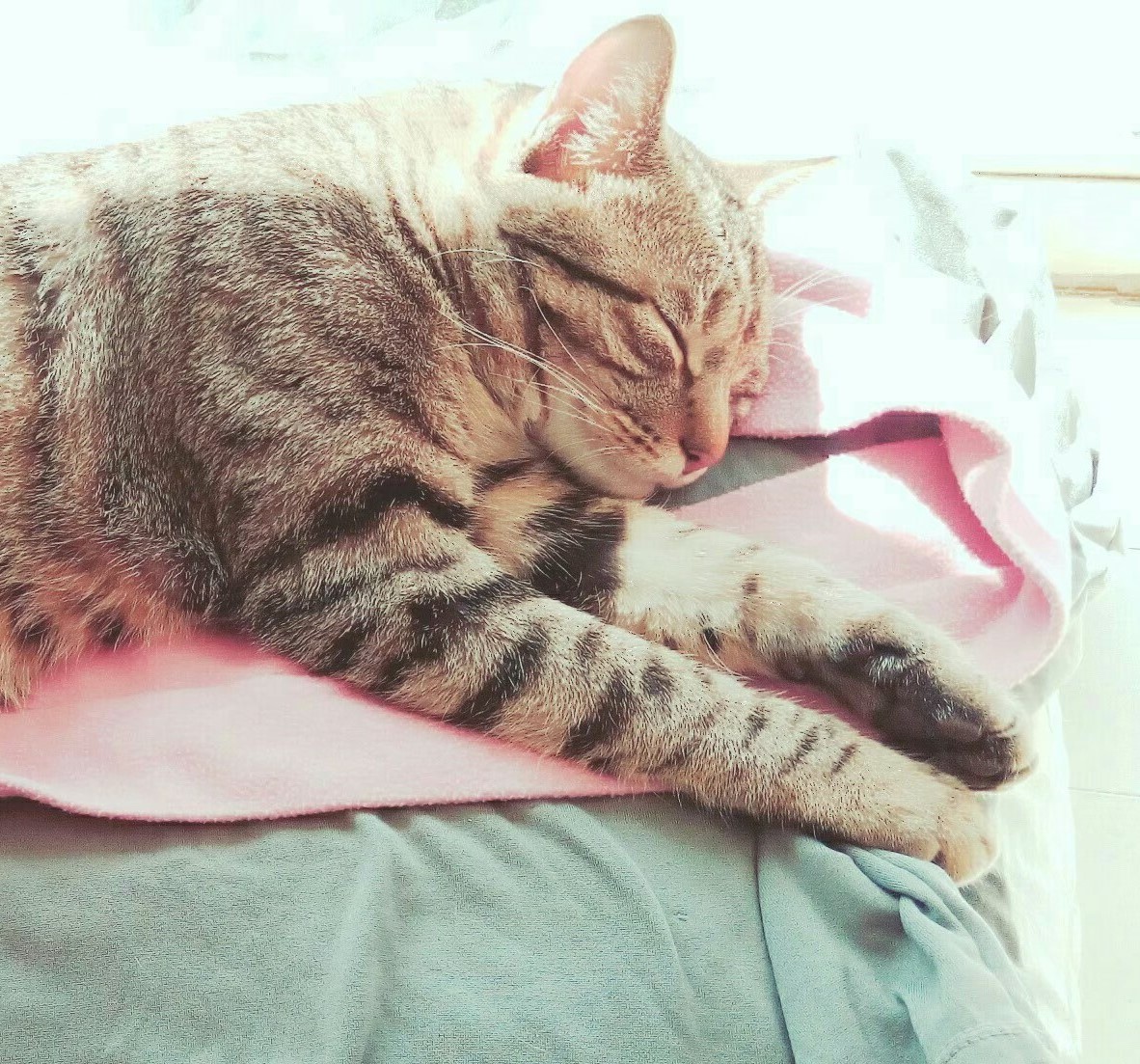
Course
可以參考我的課程簡報:
Python IntroductionIntroduction
直譯式語言
- 程式一行一行執行,不須像編譯語言先由編譯器編譯成機器碼
- 開發速度快、靈活度高
- 執行速度慢
動態語言 Dynamic Language
- 變數不須指定型態(int、string、double…)
- 執行時才確定變數型別
- 執行過程中變數型態可以改變
- ex:int -> string
強型別語言
- 不容忍隱性的型別轉換
- 比較嚴謹,方便 debug
- 強型別和弱型別不是二元論,有程度差異
Python 是強型別範例:
排版
- 縮排很重要!
- Python 不用 Block
{ }
- 排版在 Python 中非常重要
- 使用 tab,而非空白
- 不能將 tab 與空白混用
Installation
https://www.python.org/downloads/
推薦選擇加入 python.exe 到 PATH 裡,方便系統知道要到哪條路徑底下找執行檔
檢查是否下載成功,可以打開 cmd 輸入
會顯示你所下載的 Python 當前版本1
python -- version
Syntax
Hello World!
- 新增一個 main.py 檔案測試
程式碼範例
1 | print("Hello World!") |
cmd 輸出如下
變數型態
- Integer
- Bool
- String
- “string” 或 ‘string’ 都可以,只是不能混用
- Float
- 沒有 char、double
程式碼範例
1 | a = 123 # int |
cmd 輸出如下
List
- 很像 Array 的概念
- 一個 List 可以存不同型態的資料
- Index 可以是負的
- 切割 slice 非常方便
- 取某段資料
程式碼範例
1 | listTest = [1, 2.3, ⁿLAVIⁿ] # [1, 2.3, 'LAVI'] |
cmd 輸出如下
Reverse with List
- listTest = [1, 2, 3, 4, 5]
- 在不使用迴圈的前提輸出:[5, 4, 3, 2, 1],可以這樣寫
程式碼範例
1 | listTest = [1, 2, 3, 4, 5] |
cmd 輸出如下
Basic I/O
Input
- 輸入用
Input()
,括號內可以輸出內容 - 若要一次吃多個輸入,可以用
split()
- 括號內為隔開內容,常見有空白或逗號
程式碼範例
1 | a = input('input string: ') |
cmd 輸出如下
Output
- 輸出用
print()
- 自動換行
- 取消換行使用
end = ''
- Literal String Interpolation(f-string)
- Float:
f{value : width.precisionf}
- Others:
f{value : width}
- Float:
程式碼範例
1 | print(123) # 123\n |
cmd 輸出如下
{b:.6f} 輸出是 1.234568,因為會四捨五入的進位
Operators
- +、-、*、/、%
- ** 次方
- // 整除
- =
- +=、-=、/=、//=…
- 沒有 ++ 或 - -
Condition
- if
- elif
- else
程式碼範例
1 | x = int(input('Enter a number: ')) |
cmd 輸出如下
for Loop
- range(start, end, step)
- default: start = 0, step = 1
- [start, end)
- range(10)
- 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
- range(1, 9, 3)
- 1, 4, 7
程式碼範例
1 | a = [5, 4, 1, 2, 3] |
cmd 輸出如下
while Loop
程式碼範例
1 | a = 0 |
cmd 輸出如下
Function
程式碼範例
1 | def add(a, b = 0, c = 0) |
cmd 輸出如下
Class
程式碼範例
1 | class calculator(): |
cmd 輸出如下
heap
heapq 官方文件
可以在 python 直接實作 heap
建立一個 heap 可以使用 list 初始化為 []
heapq.heappush(heap, item)
heapq.heappop(heap)
heapq.heapify(x)
在線性時間內將 list x 轉為 heap
用法範例
LeetCode 786. K-th Smallest Prime Fraction
1 | class Solution(object): |
Reference
- 靜態語言 / 動態語言、強型別 / 弱型別
- 瀚丰學長的深度學習課程簡報