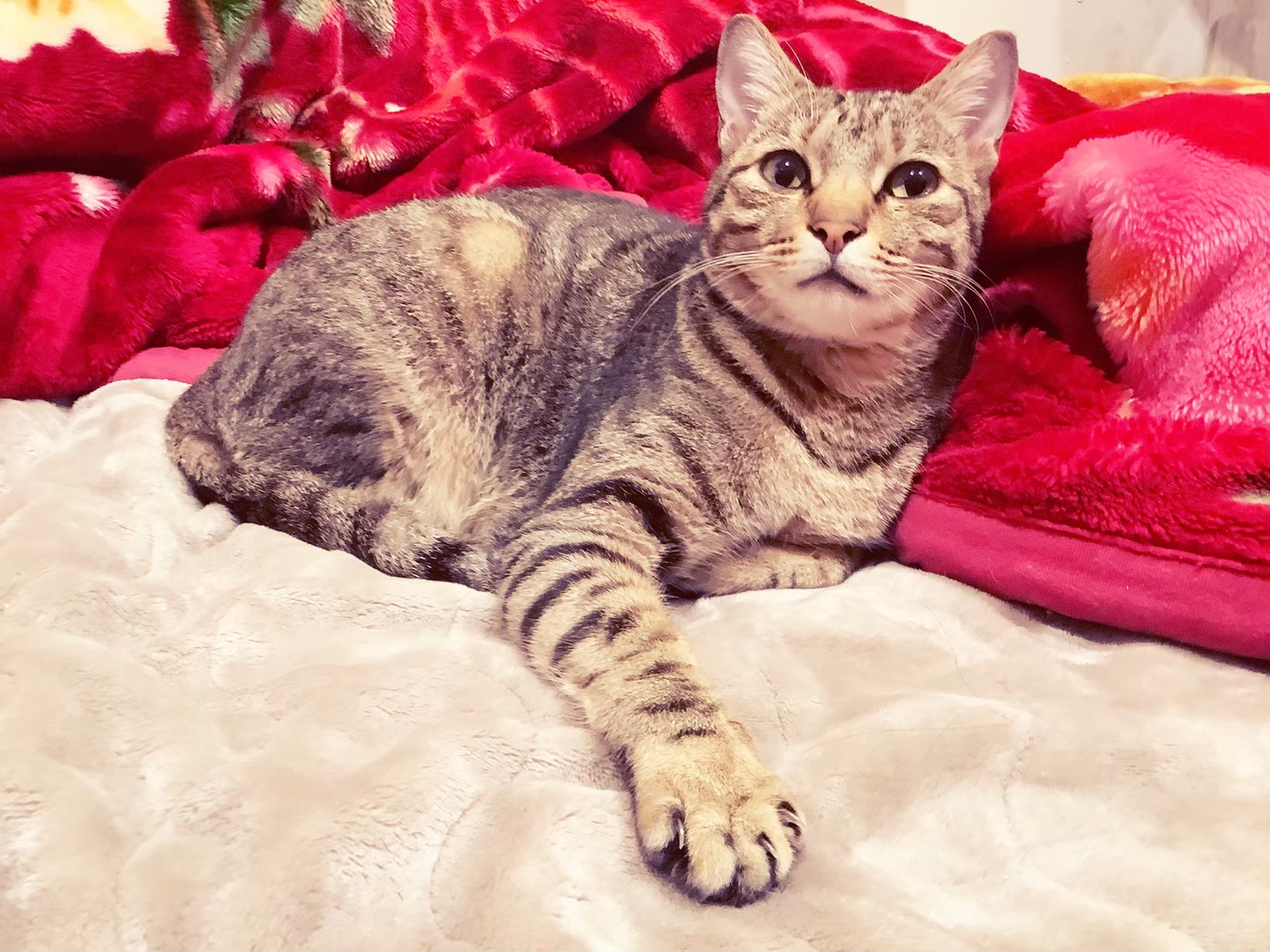
Python - PyTorch
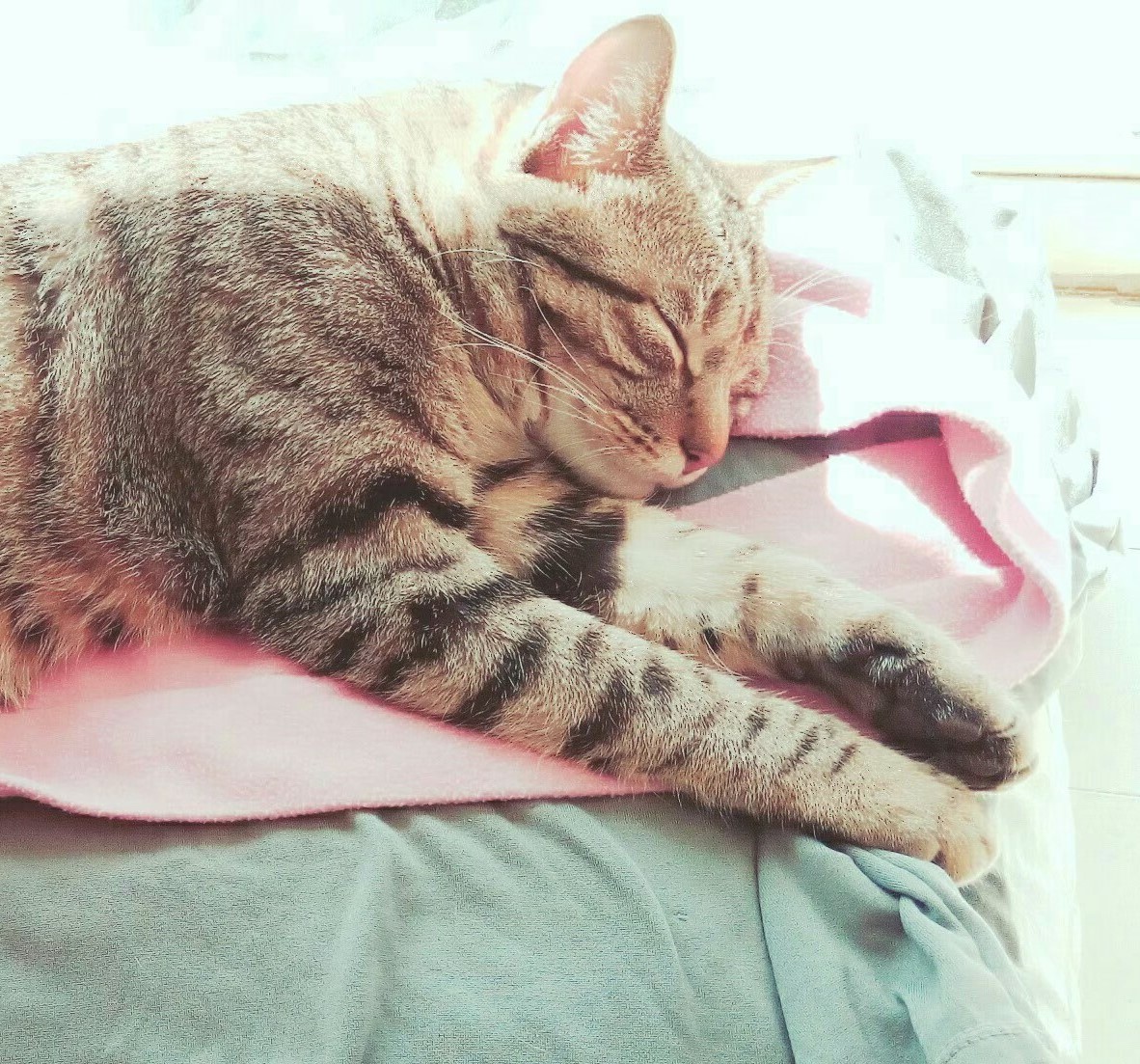
Introduction
- 將複雜的深度學習(Deep Learning,以下簡稱 DL)演算法簡化,用 PyTorch 套件內建函釋即可運算
- 專注於建構 DL 模型
- 可使用 GPU 加速模型訓練
Course
可以參考我的課程簡報:
PyTorch IntroductionWhat is PyTorch
- DL Framework
- 主要功能:
- Build Neural Network
- Loss Function & Optimizers
Syntax
- Tensor
- 高維度的向量(與 NumPy Array 相似)
- 向量相乘相加相除內積都可以在 Tensor 上做
- NumPy 用的熟的話,應該可以無痛轉接
Cuda
確定 Cuda 活著
1 | import torch |
Tensor
建立 empty 的 Tensor
1 | # 建立一維空的 tensor |
建立全部填滿指定值的 Tensor
1 | # 建立全部填滿 0 的二維 tensor |
建立全部填滿隨機值的 Tensor
1 | x = torch.rand((2, 2)) |
建立填滿自己指定值的 tensor(from List)
1 | x = torch.tensor([[1, 2], [3, 4]]) |
查看 Data Type
1 | x = torch.ones((2, 2)) |
賦予 Data Type
1 | x = torch.ones((2, 2), dtype=torch.int64) |
Data Size
1 | x = torch.ones((2, 2)) |
Tensor 和 NumPy 的轉換
1 | x = torch.ones((1, 3)) |
1 | # 轉換後記憶體位置會相同,因此資料處理時會同步!!! |
使用 GPU
1 | # 理論上在 GPU 的運算會比用 CPU 快很多 |
1 | # 算術運算必須同時在 CPU 或同時在 GPU 才能進行 |
Gradient
- 梯度
- 在建立需要更新的數值時
- 將 requires_grad 設為 True
- 開啟後計算就會自動產生 Backward Function
1 | # 在建立需要更新的數值時,將 requires_grad 設為 True |
- 例題:
- y = x + 2 且 z = y * y
- 若想計算當 x = 5 時,z 所累積的梯度,該如何計算 ?
數學算法,手會這樣算:
程式的話,要這樣寫:
1 | x = torch.tensor([5.], requires_grad=True) |
停止Gradient累計
- 如何停止 Gradient 累計 ?
- 當更新神經網路權重時,不需要產生 Gradient
- 有三種方法:
- 直接關閉,將 requires_grad_ 設為 False
- 使用 detach,會複製一份(預設requires_grad 為 False)
- with torch.no_grad(),不會對 requires_grad 產生改變,但運算時不累積梯度
1 | # 1. 直接關閉,將 requires_grad_ 設為 False |
1 | # 2. 使用 detach,會複製一份(預設requires_grad 為 False) |
1 | # 3. with torch.no_grad(),不會對 requires_grad 產生改變,但運算時不累積梯度 |
清空 Gradient
- Pytorch 的 gradient 會累加,每次用完都需要清空 gradient
- x.grad.zero_()
1 | # Pytorch 的 gradient 會累加,每次用完都需要清空 gradient |
Module
- Model 模型
- 用模型建網路
- Optimizer 優化器/最佳化器
- Gradient Descent 梯度下降的方式
- Adam、SGD… 之類的
- Criterion 評分標準
- Loss Function
Model 模型
- 依序建立模型的每層內容
- 設定模型 forward 的順序
1 | import torch.nn as nn |
Optimizer 優化器/最佳化器
- Gradient Descent 梯度下降的方式
- Adam、SGD… 之類的
- 更新權重的方式
Criterion 評分標準
- Loss Function
- Mean Square Error、CrossEntropy、Binary Cross Entropy… 之類的
Modle Training
- Model 設定為 Train 模式
- Forward
- Calculate Loss
- Backward
1 | # 初始化 X, Y 為 3 * 1 的矩陣 |
Model Testing
- Model 設定為 eval 模式( evaluation 評估模式)
- 停止 gradient 累計
1 | # 這邊的 model.fc 是沿用剛才 Build Model 的 function(客家人) |
Reference
- 上屆助教們的簡報 <(_ _)>
- 黃貞瑛老師的課程與簡報